老师的方法:
select job_id,sum(salary),sum(decode(department_id,20,salary))"Dep20",sum(decode(department_id,50,salary))"Dep50",sum(decode(department_id,80,salary))"Dep80" ,sum(decode(department_id,90,salary))"Dep 90"from employees group by job_id order by sum(salary);
结果:
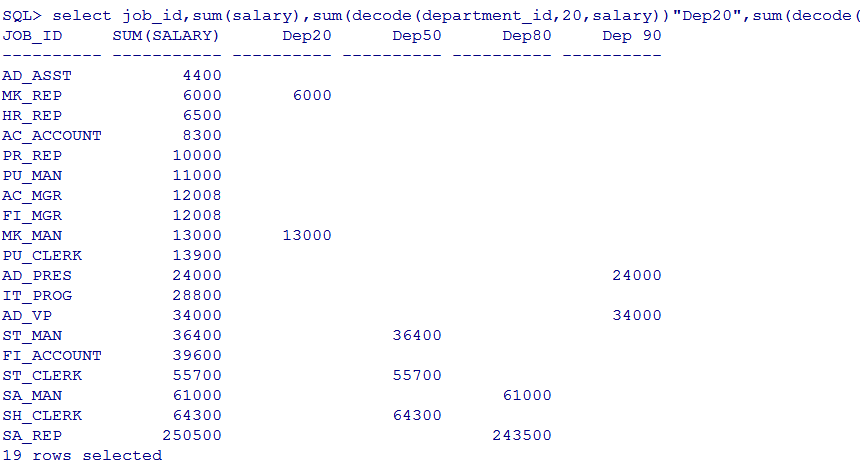
我的方法:
select job_id, sum(salary), decode(department_id, 20, sum(salary)), decode(department_id, 50, sum(salary)), decode(department_id, 80, sum(salary)), decode(department_id, 90, sum(salary)) from employees e group by job_id, department_id order by sum(salary);
结果:
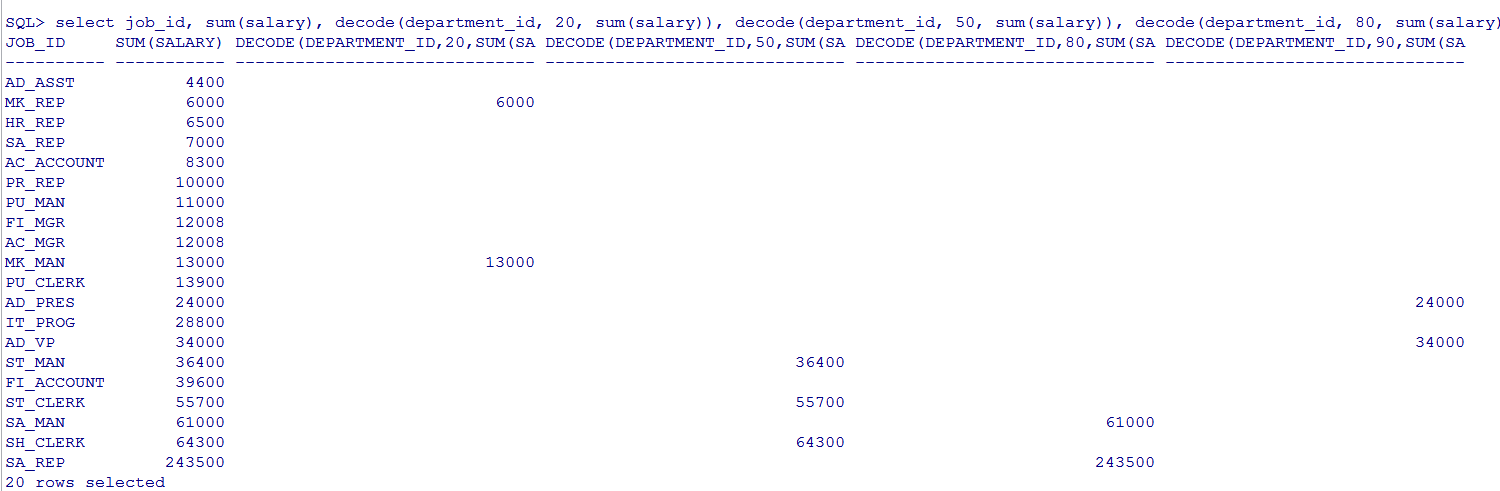
我与老师的区别是:
老师是sum里面放decode,后面不用加department_id的分组
我的是decode里面放sum,后面需要加department_id的分组
两组结果的区别是
我的返回的是20个结果,
老师返回的是19个结果
这两个的区别我没有搞清楚,老师可以解惑一下么?