老师,我的这个代码可以成功插入数据,可是在执行成功前会显示异常是怎么回事?
public class JdbcUtil {
private static String driver;
private static String url;
private static String username;
private static String password;
static {
ResourceBundle bundle=ResourceBundle.getBundle("jdbc");
driver=bundle.getString("driver");
url=bundle.getString("url");
username=bundle.getString("username");
password=bundle.getString("password");
try {
Class.forName(driver);
} catch (Exception e) {
e.printStackTrace();
}
}
public static Connection getConnection(){
Connection conn=null;
try {
conn= DriverManager.getConnection(url,username,password);
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
private static void closeStatement(Statement state){
if(state != null){
try {
state.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
public static void closeConnection(Connection conn){
if(conn != null){
try {
conn.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
public static void closeResource(Statement state,Connection conn){
closeStatement(state);
closeConnection(conn);
}
}
public class JDBCTest {
public void insertData(String department_name,int location_id){
Connection conn=null;
Statement state=null;
try {
conn=JdbcUtil.getConnection();
conn= DriverManager.getConnection("jdbc:mysql://localhost:3306/zf?useUnicode=true&characterEncoding=utf8","root","zfroot");
String sql="insert into department value(default ,'"+department_name+"',"+location_id+")";
state=conn.createStatement();
int flag=state.executeUpdate(sql);
System.out.println(flag);
} catch (Exception e) {
e.printStackTrace();
}finally {
JdbcUtil.closeResource(state,conn);
}
}
public void updateData(String department_name,int location_id,int department_id){
Connection conn=null;
Statement state=null;
try {
conn=JdbcUtil.getConnection();
String sql="update department set department_name='"+department_name+"',location_id="+location_id+" where department_id="+department_id+";";
state=conn.createStatement();
int flag=state.executeUpdate(sql);
System.out.println(flag);
} catch (Exception e) {
e.printStackTrace();
}finally {
JdbcUtil.closeResource(state,conn);
}
}
public static void main(String[] args) {
JDBCTest jdbcTest=new JDBCTest();
// jdbcTest.updateData("策划部",6,3);
jdbcTest.insertData("经理部",7);
}
}
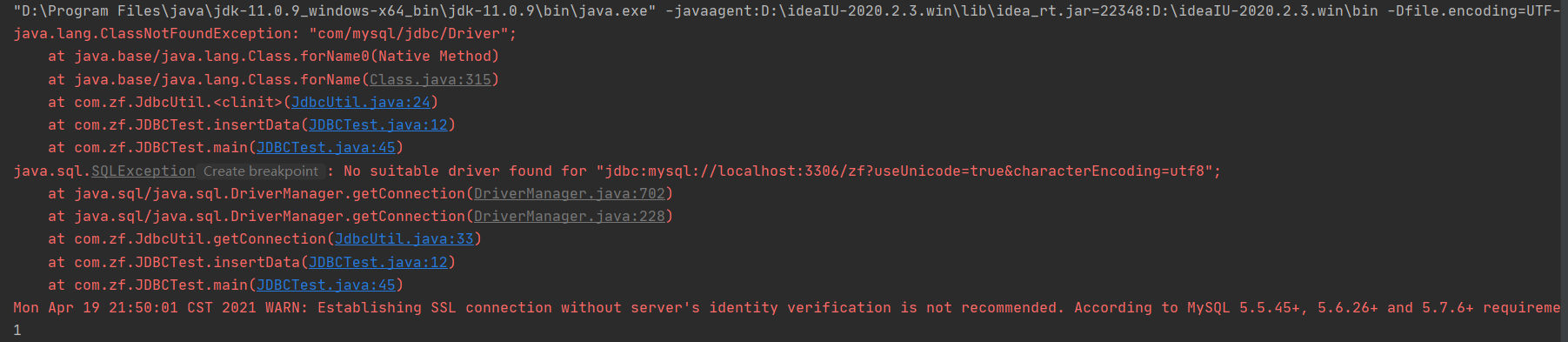
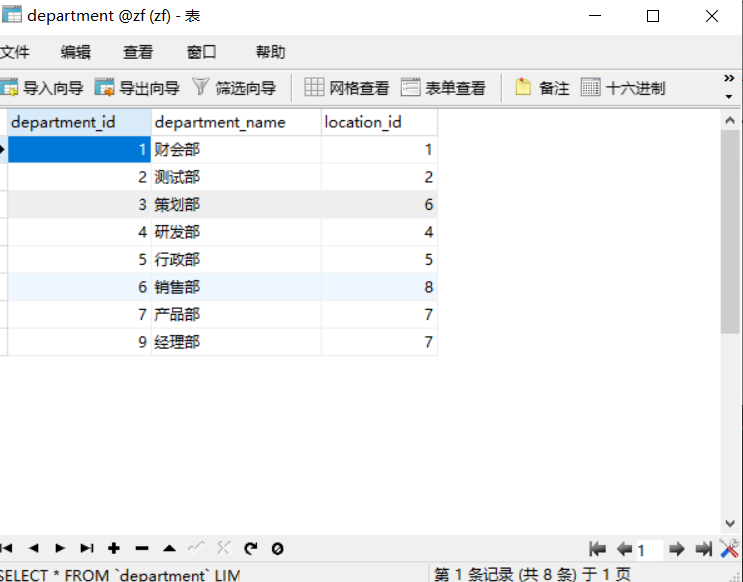