问题: Cannot forward after response has been committed问题的解决?
问题描述:将所有的Servlet整合到一个servlet当中报错(分开的话,没有问题,合并的话,问题就出现了)。
点击查询新闻后,并不能成功跳转页面,大部分情况只会在servlet中停留。但有时候退出,再次点击查询新闻后,则能够成功进入页面,但是控制台任然报错。
问题应该出现在整合后的servlet这个方法中了。
private void doQueryNewsServlet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
NewsService ns = new NewsServiceImpl();
List<News> list = ns.queryAllNews();
System.out.println("失败了");
//对结果进行处理
request.setAttribute("list", list);
try {
request.getRequestDispatcher("queryAllNews.jsp").forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
问题二:request改成session会报 无法反序列化 ,这是什么原因导致的?
如果将上述代码中的request.setAttribute("list", list);
改成
request.getSession().setAttribute("list", list);
接收方也改成了session,运行时会报 无法发序列化list
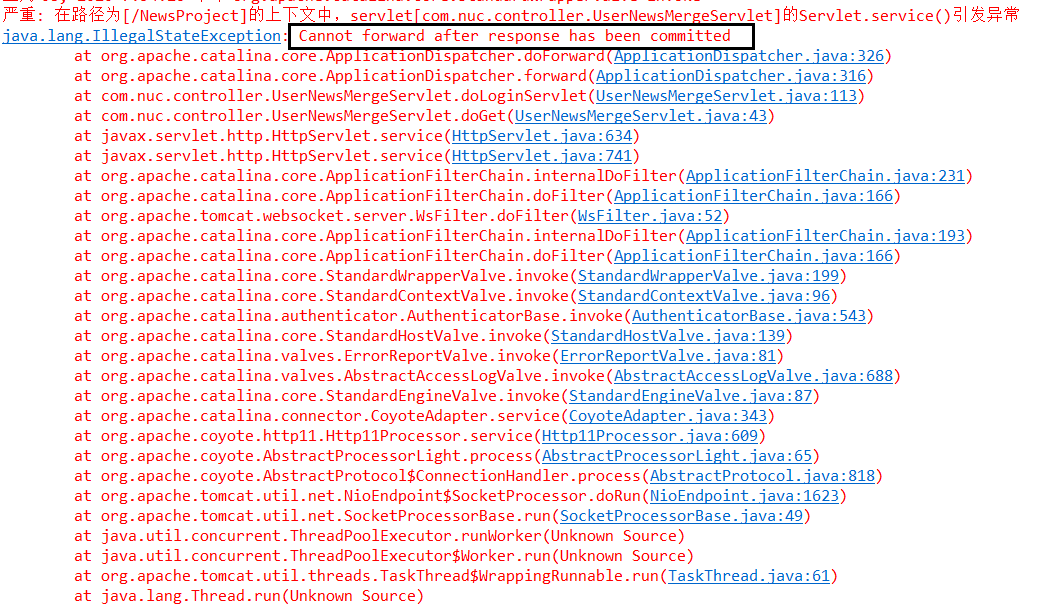