我在使用封装的通用的查询方法时,regtime查询结果是null的(regtime是Timestamp类型),但是我用rs.getTimestamp("REGTIME"),不是通用的方法查询的时候可以查得到。不知道为什么
protected <T> T queryOne(Class<T> cls,String sql,Object... params) {
Connection conn = DBUtil.getConn();
PreparedStatement pstmt = DBUtil.getPstmt(conn, sql);
DBUtil.bindParam(pstmt, params);
ResultSet rs = null;
try {
rs = pstmt.executeQuery();
ResultSetMetaData metData = rs.getMetaData();
if(rs.next()) {
T bean = cls.newInstance();
for(int i=0;i<metData.getColumnCount();i++) {
BeanUtils.setProperty(bean, metData.getColumnLabel(i+1).toLowerCase(), rs.getObject(i+1));
}
return bean;
}
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}finally {
DBUtil.close(rs, pstmt, conn);
}
return null;
}
返回结果:

protected User queryOne2(String sql,Object... params) {
Connection conn = DBUtil.getConn();
PreparedStatement pstmt = DBUtil.getPstmt(conn, sql);
DBUtil.bindParam(pstmt, params);
ResultSet rs = null;
try {
rs = pstmt.executeQuery();
ResultSetMetaData metData = rs.getMetaData();
if(rs.next()) {
User bean = new User();
bean.setId(rs.getInt("ID"));
bean.setUname(rs.getString("UNAME"));
bean.setRegTime(rs.getTimestamp("REGTIME"));
bean.setIntegrate(rs.getInt(4));
bean.setSex(rs.getString("SEX"));
return bean;
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
DBUtil.close(rs, pstmt, conn);
}
return null;
}
返回结果:

javabean:
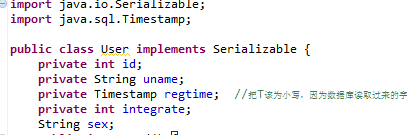