import pymysql
class Mydb:
config={
"host":"localhost",
"user":"root",
"password":"root",
"db":"music_project"
}
def __init__(self):
self.connection=pymysql.connect(**Mydb.config)
self.cursor=self.connection.cursor()
def close(self):
if self.connection:
self.connection.close()
if self.cursor:
self.cursor.close()
#执行插入 修改 删除
def exeDML(self,sql,*args):
try:
#执行sql
count=self.cursor.execute(sql,args)
#提交事务
self.connection.commit()
return count
except Exception as e:
print(e)
if self.connection:
self.connection.rollback()
finally:
self.close()
def query_one(self,sql,*args):
try:
self.cursor.execute(sql,args)
#获取结果集
return self.cursor.fetchone()
except Exception as e:
print(e)
finally:
self.close()
def query_all(self,sql,*args):
try:
self.cursor.execute(sql,args)
#获取结果集
return self.cursor.fetchall()
except Exception as e:
print(e)
finally:
self.close()
if __name__ == "__main__":
dbutil=Mydb()
from dbutil import Mydb
class MyService:
def login(self,uname,password):
sql="select * from t_user where uname=%s and password=%s"
user=Mydb.query_one(sql,uname,password)
if user:
return True
else:
return False
#登陆界面
from Myservice import MyService
if __name__=="__main__":
uname=input("请输入用户名:")
password=input("请输入密码:")
myservice=MyService()
if myservice.login(uname,password):
print("登陆成功")
else:
print("登陆失败")
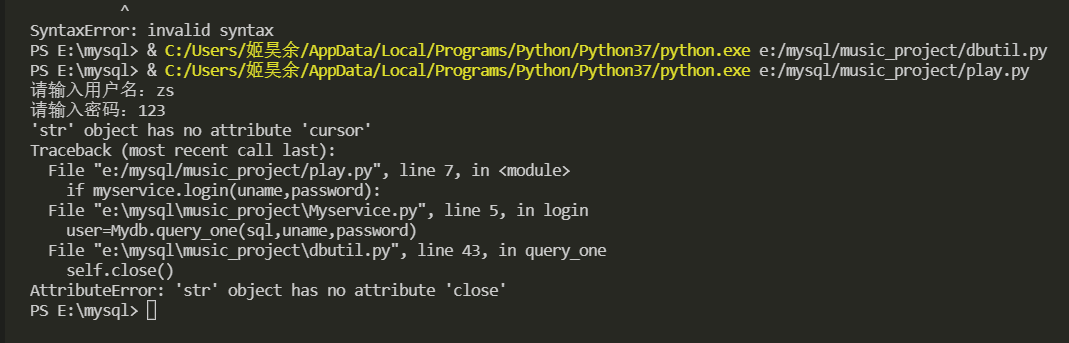
老师 你帮我看下我这个 是哪里有问题