老师好,请看一下:
问题:无法获取Goods类的brand属性
商品类:
package cn.sxt.thread;
public class Goods {
private String name;
private String brand;
private boolean flag;//标志位,用来表示是否存在商品 flag = true表示有商品
public Goods(String name, String brand) {
super();
this.name = name;
this.brand = brand;
}
public Goods() {
super();
// TODO Auto-generated constructor stub
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public boolean isFlag() {
return flag;
}
public void setFlag(boolean flag) {
this.flag = flag;
}
//同步的用来获取商品的方法,用来避免数据错乱,线程通信notify和wait用来防止数据重复
public synchronized void get() {
if (!flag) {//相当于flag = false没有商品,消费者等待
try {
super.wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
//消费者线程被唤醒,开始消费
System.out.println("消费者消费:"+this.getBrand()+"\t"+this.getName());
//通知生产者生产
super.notify();
flag = false;//表示没有商品了
}
//同步方法用来生产商品,同步用来避免数据错乱,线程通信notify/wait用来防止数据重复生产或者消费
public synchronized void set(String Brand,String name) {
if (flag) {//表示有商品,生产等待
try {
super.wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
//生产者线程被唤醒,开始生产
this.name = name;
this.brand = brand;
System.out.println("生产者生产:"+this.getBrand()+"\t"+this.getName());
//通知消费者消费
super.notify();
flag = true;//表示 有商品
}
}
生产者:
package cn.sxt.thread;
public class Producer implements Runnable {
private Goods g;
public Producer(Goods g) {
super();
this.g = g;
}
public Producer() {
super();
// TODO Auto-generated constructor stub
}
@Override
public void run() {
// TODO Auto-generated method stub
for(int i=0;i<10;i++) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
if (i%2==0) {//偶数
g.set("旺仔", "小馒头");
}else {
g.set("哇哈哈", "矿泉水");
}
}
}
}
消费者:
package cn.sxt.thread;
public class Consumer implements Runnable {
private Goods g ;
public Consumer(Goods g) {
super();
this.g = g;
}
@Override
public void run() {
// TODO Auto-generated method stub
for(int i=0;i<10;i++) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
g.get();
}
}
}
测试类:
package cn.sxt.thread;
public class TestGoods {
public static void main(String[] args) {
//创建 商品类
Goods g = new Goods();
//创建生产者,消费者线程
Producer p = new Producer(g);
Consumer c = new Consumer(g);
//启动线程
new Thread(p).start();
new Thread(c).start();
}
}
运行效果截图:
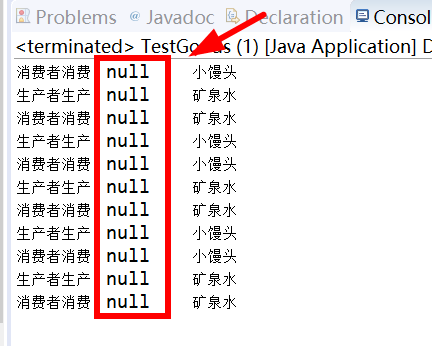