——————————————————————————————
老师请问一下,我的代码,没进行线程同步,却怎么也试不出品牌与商品错乱的情况?试了好几次了
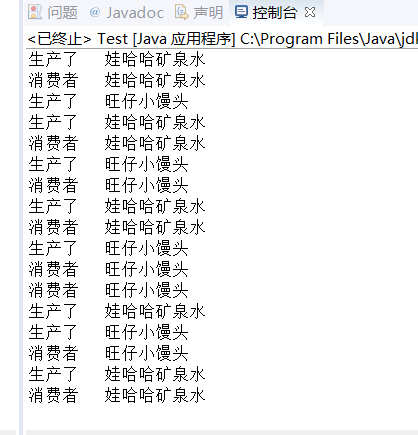
package com.jingdong.www;
//商品类
public class Goods {
//品牌
private String brand; //通过赋值,实现多种商品简单点
private String commodity;
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getCommodity() {
return commodity;
}
public void setCommodity(String commodity) {
this.commodity = commodity;
}
public Goods(String brand, String commodity) {
super();
this.brand = brand;
this.commodity = commodity;
}
public Goods() {
super();
}
}
package com.jingdong.www;
//生产者类 线程才能同时嘛
public class Producer implements Runnable{
Goods producer;
public Producer(Goods producer) {
super();
this.producer = producer;
}
@Override
public void run() {
// TODO 自动生成的方法存根
for(int i=1;i<=10;i++) { //生产10次
if(i%2==0) {//双数生产娃哈哈
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
producer.setBrand("娃哈哈");
producer.setCommodity("矿泉水");
}else {//如果是单数生产旺仔小馒头
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
producer.setBrand("旺仔");
producer.setCommodity("小馒头");
}
//生产什么打印什么
System.out.println("生产了 "+producer.getBrand()+""+producer.getCommodity());
}
}
}
package com.jingdong.www;
//顾客类
public class Customer implements Runnable{
//商品实例
private Goods customer;
public Customer(Goods customer) {
super();
this.customer = customer;
}
@Override
public void run() {
// TODO 自动生成的方法存根
for(int i=1;i<=10;i++) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
System.out.println("消费者 "+customer.getBrand()+customer.getCommodity());
}
}
}
package com.jingdong.www;
public class Test {
public static void main(String[] args) {
Goods goods=new Goods();
new Thread(new Producer(goods)).start();
new Thread(new Customer(goods)).start();
}
}