老师,您好,我在这里遇到了问题,问题如下
问题描述:在进行Reseponse封装后,服务器能启动起来,但在html文档里面输入内容后服务器反馈异常。
相关代码:
package com.like.server;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import com.like.util.IOCloseUtil;
public class Response {//响应
private StringBuilder headInfo;//响应头
private StringBuilder content;//响应内容
private int length;//响应内容长度
//流
private BufferedWriter bw;
//两个常量,换行和空格
private static final String CRLF="\r\n";
private static final String BLANK=" ";
//构造方法
public Response() {
headInfo=new StringBuilder();
content=new StringBuilder();
}
public Response(OutputStream os) {
this();//调用本类的无参构造方法
try {
bw=new BufferedWriter(new OutputStreamWriter(os,"utf-8"));
} catch (UnsupportedEncodingException e) {
headInfo=null;
}
}
//构造正文部分
public Response print(String info) {//不换行的
content.append(info);
try {
length+=info.getBytes("utf-8").length;
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return this;
}
public Response println(String info) {//换行的
content.append(info).append(CRLF);
try {
length+=info.getBytes("utf-8").length;
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return this;
}
//构造响应头
private void createHeadInfo(int code) {
headInfo.append("HTTP/1.1").append(BLANK).append(code).append(BLANK);
switch(code) {
case 200:
headInfo.append("OK");
break;
case 500:
headInfo.append("SERVER ERROR");
break;
default:
headInfo.append("NOT FOUND");
break;
}
headInfo.append(CRLF);
headInfo.append("Content-Type:text/html;charse=utf-8").append(CRLF);
headInfo.append("Content-Length:"+length).append(CRLF);
headInfo.append(CRLF);
}
public void pushToClient(int code) {
if(headInfo==null) {
code=500;
}
try {
//调用本类中的响应头
this.createHeadInfo(code);
bw.write(headInfo.toString());
bw.write(content.toString());
bw.flush();
this.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void close() {
IOCloseUtil.closeAll(bw);
}
}
Server:
public class Server {//服务器,用于启动和停止服务器
private ServerSocket server;
public static void main(String[] args) {
Server server=new Server();
server.start();
}
public void start() {
this.start(8888);
}
public void start(int port) {
try {
server=new ServerSocket(port);
this.receive();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void receive() {
//监听
try {
Socket client=server.accept();
/*
//获取用户的请求
InputStream is=client.getInputStream();
byte[] buf=new byte[20480];
int len=is.read(buf);
System.out.println(new String(buf,0,len));*/
//封装请求信息
Request req=new Request(client.getInputStream());
req.show();
Response rep=new Response(client.getOutputStream());
Servlet servlet=WebApp.getServlet(req.getUrl());
int code=200;
if(servlet==null) {
code=404;
}
//调用Servlet中的服务方法
try {
servlet.service(req,rep);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
rep.pushToClient(code);
IOCloseUtil.closeAll(client);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void stop() {}
}
HTML
<html>
<head>
<title>我是第一个html</title>
</head>
<body>
<h1>hello world!</h1>
<form action="http://localhost:8888/log" method="get">
<p>用户名:<input type="text" id="uname" name="username" /></p>
<p>密  码:<input type="password" id="pwd" name="password" /></p>
<p>兴趣爱好:<input type="checkbox" name="hobby" value="ball"/>球
<input type="checkbox" name="hobby" value="read"/>读书
<input type="checkbox" name="hobby" value="paint"/>画画</p>
<p><input type="submit" value="登录"/></p>
</form>
</body>
</html>
异常截图如下:
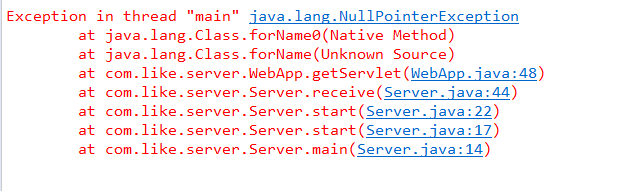
在这之前的检测都是正常的。
源代码:
http_server6.zip