看完了这节课的视频以后,总结了下面的内容:
这节课的视频与上次课的视频不同的点在
上次课最后实现的多线程是 多个客户端访问一个服务器的情况,为了让服务器处理多个客户端,使用循环开启多个线程,为每一个客户端开启一个线程来处理服务器端接收信息及相应信息的操作。
while(true){
Socket socket=server.accept();//接受多个客户端的连接
ServerThread st=new ServerThread(socket);
new Thread(st).start();
}
这次课的多线程是客户端的多线程,是单个客户端和单个服务器端之间,单个客户端想跟单个服务器端多次聊天,如果是单次聊天,按视频里最开始的写法(没有多线程的),客户端就只能先,通过BufferedReader包装键盘录入发送信息,后获取响应,并且只有一次聊天的机会,socket就结束了。
为了解决这两个问题:
1)客户端想多次聊天
2)客户端能不能不要总是先发送,后接受信息,能主动接受信息吗?
解决:
1) 循环,循环发送信息,循环读取信息,就可以多次聊天了
2)怎么能决定先发送还是先接受,如果写成以前死的代码,就是先发送后接受,想先接受怎么办,多线程,建立一个发送线程,一个接受线程,让2个线程开启,cpu不断的切换2个线程,有内容发送到客户端来,就主动去接受,就可以先接受了。这边键盘录入,用户想发送的时候按下回车,就可以向输入流中写数据,创造输入的字符串,有内容想发送到服务端,就会触发发送线程。
我分析的视频中的代码:
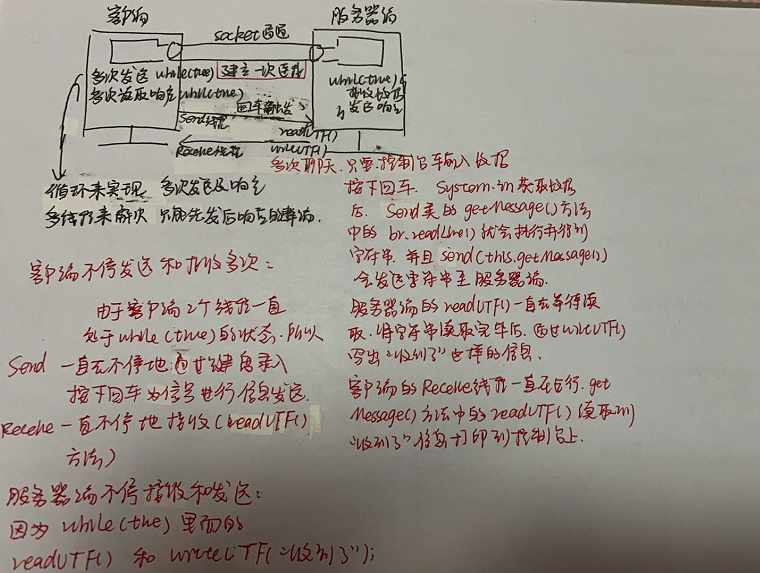
一个客户端 一个服务端
服务端只接受一次的socket对象,后面的多次聊天都是因为
客户端一直开启线程,等待用户键盘录入的回车,从而发送数据,
服务器端接收到数据就一直执行while(true),不停的readUTF() 和writeUTF("收到了");
至于我看到有同学问为什么服务器端能一直读取,我想是因为while(true)里面的readUTF()在不停执行,只要在流里读到东西就会读取出来,在流里东西可用,检测到流末尾或者抛出异常前,此方法一直阻塞,等待读取的字符串。这里需要老师验证一下我说的,是不是我分析的这个原因******。
还有一个问题******,就是在客户端线程类:Send类的send方法中写出字符串的时候,为什么要flush一下。
我猜是因为:这里也需要老师解答一下:
1)每次写出之后flush是为了提高效率,如果一直不flush,缓冲区如果满了就会造成缓冲区堵塞
2)视频里的案例没有关闭流,关闭流的操作里会刷新,但是没有关闭流,一直开着,所以在这里刷新一下。
ps:
什么时候字节流需要刷新:
如果你的流入数据不是特别多,可以都操作结束了关流时写入,那么如果你的数据特别多,你把他们全都放到流中就会占用内存吧,如果占用够多就会死机吧?然后结果你明白了!你读取数据失败了!或者説你担心万一死机了数据没有保存不是很坑!所以你可以选择什么时候刷新下
所以当传入的数据特别大,又担心什么时候会突然停止的时候,字节流可以在某些地方刷新一下。
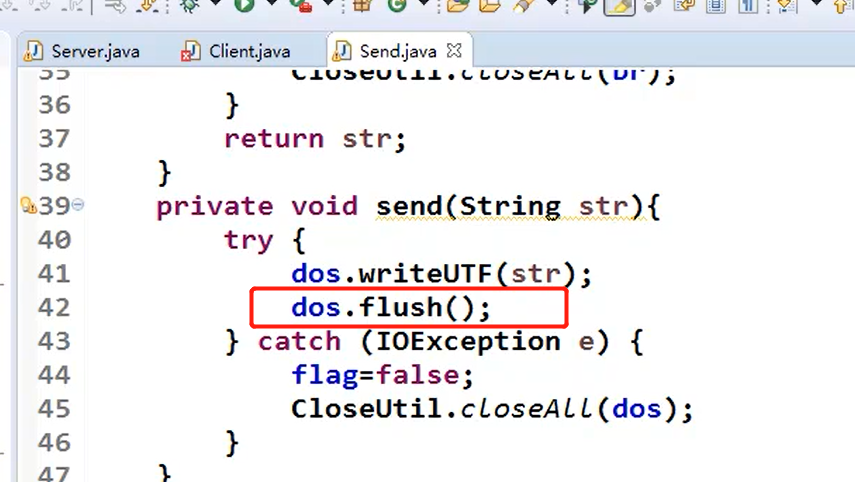
以上提到的两个地方都需要老师解答一下(带******号的),还有提到的其他地方如果有不太对的地方,也希望老师指导一下,谢谢!