老师Scanner为什么会报空指针异常
package internet;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class TwoSever {
public static void main(String[] args) {
ServerSocket serverSocket = null;
try {
serverSocket = new ServerSocket(8888);
System.out.println("服务端启动,监听端口8888");
Socket socket = serverSocket.accept();
System.out.println("连接成功!");
Thread t= new Thread(new ServiceRead(socket));
Thread t1= new Thread(new ServiceWrite(socket));
t1.start();
t.start();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
}
}
}
//接收消息的
class ServiceRead implements Runnable{
private Socket socket;
public ServiceRead(Socket socket) {
this.socket=socket;
}
@Override
public void run() {
regular();
}
private void regular() {
BufferedReader bu= null;
try {
bu = new BufferedReader(new InputStreamReader(socket.getInputStream()));
while(true) {
String str=bu.readLine();
System.out.println("客户端说:"+str);
}
}catch(Exception e) {
e.printStackTrace();
}finally {
try {
if(bu != null) {
bu.close();
}
if(socket != null) {
socket.close();
}
}catch(Exception e) {
e.printStackTrace();
}
}
}
}
//sever发送消息
class ServiceWrite implements Runnable{
private Socket socket;
public ServiceWrite(Socket socket) {
this.socket=socket;
}
@Override
public void run() {
send();
}
private void send() {
Scanner scanner = null;
PrintWriter bw =null;
try {
bw= new PrintWriter(this.socket.getOutputStream());
while(true) {
String str = scanner.nextLine();
bw.print(str);
bw.flush();
}
}catch(Exception e) {
e.printStackTrace();
}finally {
try {
if(scanner != null) {
scanner.close();
}
if(bw != null) {
bw.close();
}
if(socket !=null) {
socket.close();
}
}catch(Exception e) {
e.printStackTrace();
}
}
}
}
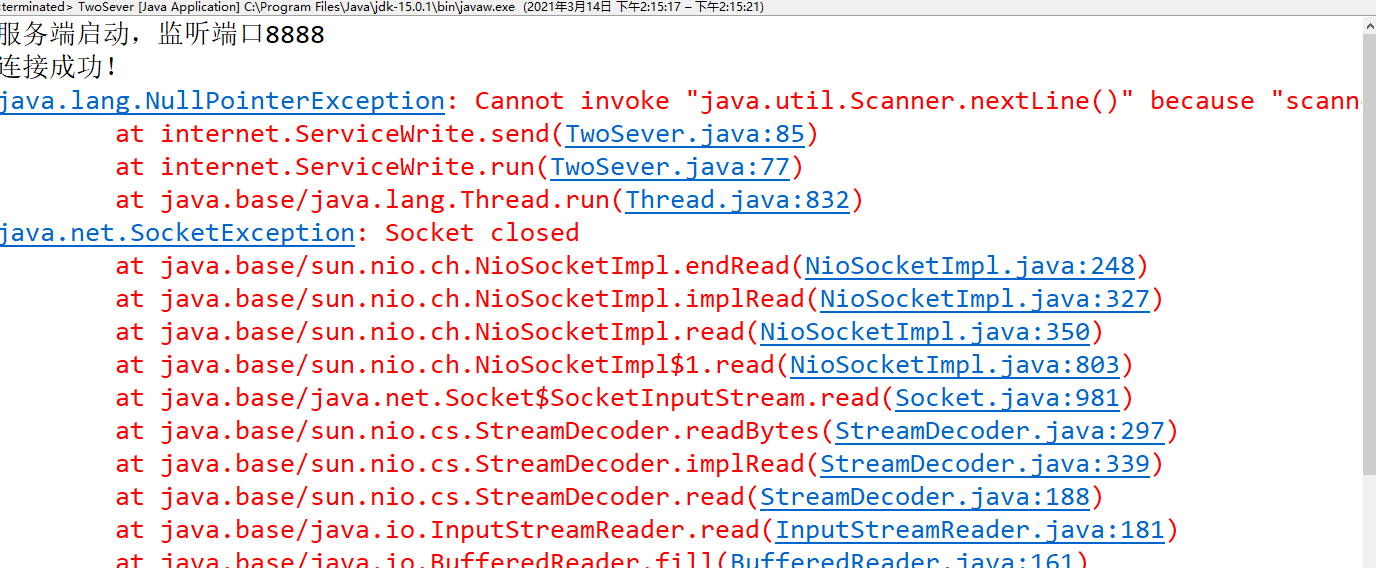