package com.bjsxt.io;
import java.io.Serializable;
import java.util.ArrayList;
/**
* <p>Title: Class403</p>
* <p>Description: 自定义对象2(将对象序列化写入文件中)</p>
* @author xiaoding
* @date Jun 10, 2020
* @version 1.0
*/
public class Class403 implements Serializable {
//功能属性
private int classs; //班级
private ArrayList<Student> a1; //学生
// private int num;
//构造方法
Class403() {
super();
}
public Class403(int classs,ArrayList<Student> a1) {
this.classs = classs;
this.a1 = a1;
}
//get、set方法
public int getClasss() {
return classs;
}
public void setA1(ArrayList<Student> a1) {
this.a1 = a1;
}
public ArrayList<Student> getA1() {
return a1;
}
public void setClasss(int classs) {
this.classs = classs;
}
//toString方法
@Override
public String toString() {
return "Class403 [classs=" + classs + ", a1=" + a1 + "]";
}
}
package com.bjsxt.io;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
/**
* <p>Title: TestClass403</p>
* <p>Description: 测试类2测试序列化和反序列化</p>
* @author xiaoding
* @date Jun 10, 2020
* @version 1.0
*/
public class TestClass403 {
//写入对象
public static void write() {
//创建ArrayList对象,写入学生信息
ArrayList<Student> a1 = new ArrayList<Student>();
a1.add(new Student(0,"张三",20));
a1.add(new Student(1,"李四",30));
a1.add(new Student(2,"王五",25));
a1.add(new Student(3,"小明",18));
ObjectOutputStream fis = null;
//创建ObjectOutputStream写入对象
try {
fis = new ObjectOutputStream(new FileOutputStream("D:/student.txt"));
fis.writeObject(new Class403(403,a1));
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
//关闭IO流
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
//读取对象
public static void read() {
//创建ObjectInputStream
ObjectInputStream fis = null;
try {
fis = new ObjectInputStream(new FileInputStream("D:/student.txt"));
//读取对象
System.out.println(fis.readObject());
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
//关闭IO流
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
//main方法
public static void main(String[] args) {
//write();
read();
}
}
首先第一次序列化对象,Class403的属性值只有两个,先写入在自读取
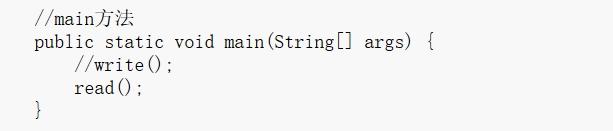
第二次,更改Class403的实例属性,
// private int num; 解除注释
输出方法还是上面图片那样,他会报错,因为版本不兼容,需要指定版本号
但是我改成这样输出就不会报错,为什么?
