老师,在29-34行中,使用接口Animal类来获取Mouse,Bird的run,fly获取不了,视频里老师也没有进行这2个参数的演示。
from flask import Flask
from flask_graphql import GraphQLView
import graphene
class Animal(graphene.Interface): #定义一个接口类
id=graphene.ID()
name=graphene.String()
class Mouse(graphene.ObjectType):
class Meta:
interfaces=(Animal,) #继承接口中的属性
run = graphene.String() #自定义属性
class Bird(graphene.ObjectType):
class Meta:
interfaces = (Animal,) #继承接口中的属性
fly = graphene.String() #自定义属性
class Query(graphene.ObjectType):
mouse=graphene.Field(Mouse) #获得Mouse属性
bird=graphene.Field(Bird) #获得Bird属性
animal=graphene.Field(Animal,type_=graphene.Int(required = True))
def resolve_mouse(self,info):
return Mouse(id=1,name='杰瑞',run='汤姆快跑呀~~')
def resolve_bird(self,info):
return Bird(id=2,name='高书博',fly='汤姆快跑呀~~')
# def resolve_animal(self,info,type_):
# if type_==1:
# return Mouse(id=3, name='狗蛋高书博', run='追唐老鸭啦~~~')
# elif type_==2:
# return Bird(id=4, name='狗子高书博', fly='跑呀~Tom来了!!')
def resolve_animal(self,info,type_):
# return Animal(id=3,name='动物') # "message": "An Interface cannot be intitialized",
if type_ == 1:
return Mouse(id= 4, name='米老鼠',run='追唐老鸭啦~~~')
else:
return Bird(id = 3,name='鹦鹉',fly = '跑呀~Tom来了!!')
if __name__=='__main__':
schema=graphene.Schema(query=Query) #查询Query
app=Flask(__name__) #创建flask对象
# /graphql:视图的URL, GraphQLView.as_view:将映射函数转换成视图函数 ,'grapql':视图名称 ,
# 把schema注册到schema方法里, graphiql=True:开启插件应用
app.add_url_rule('/graphql', view_func=GraphQLView.as_view('grapql', schema=schema, graphiql=True))
app.run()
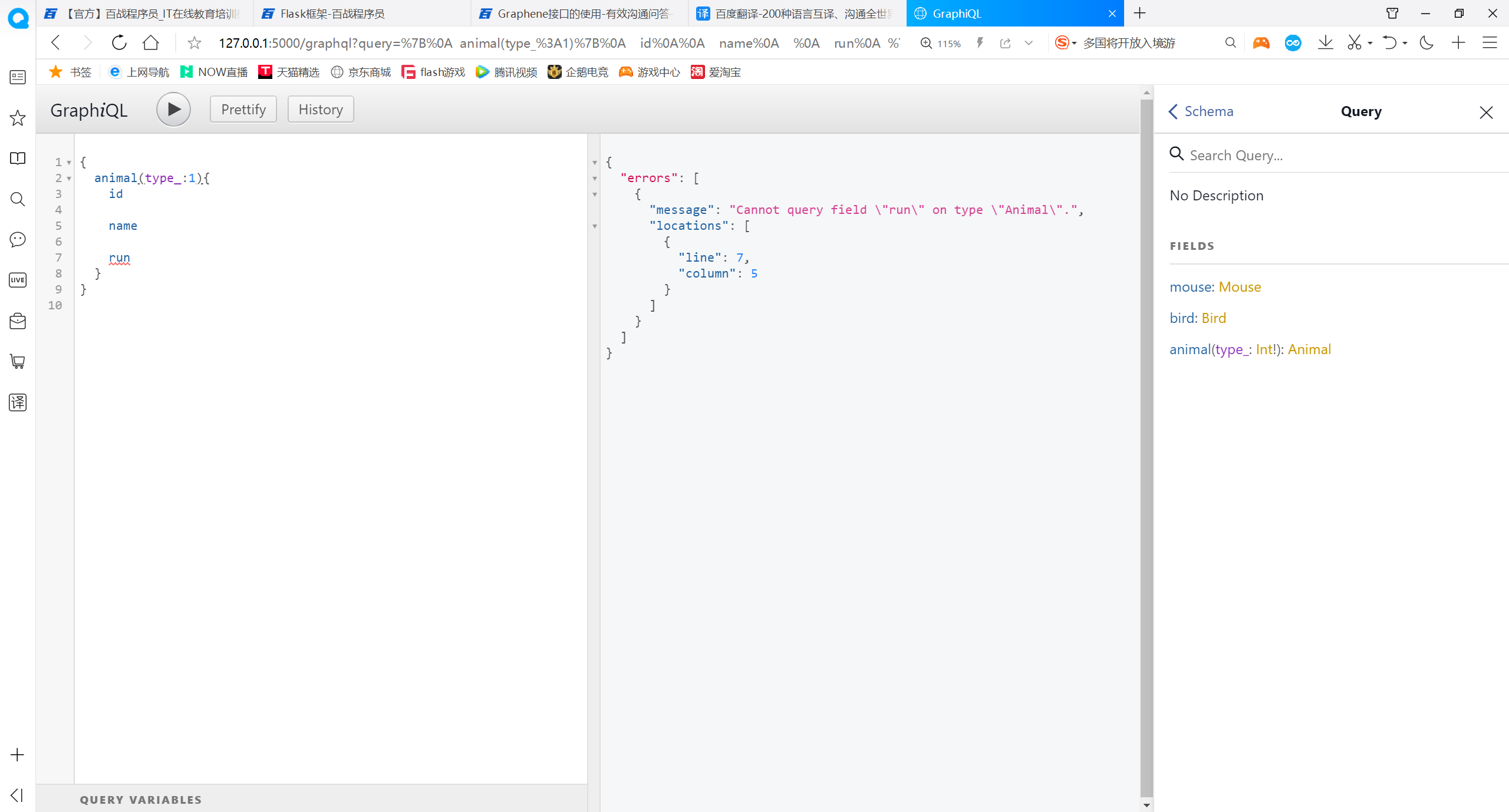