post带参数请求取不到内容
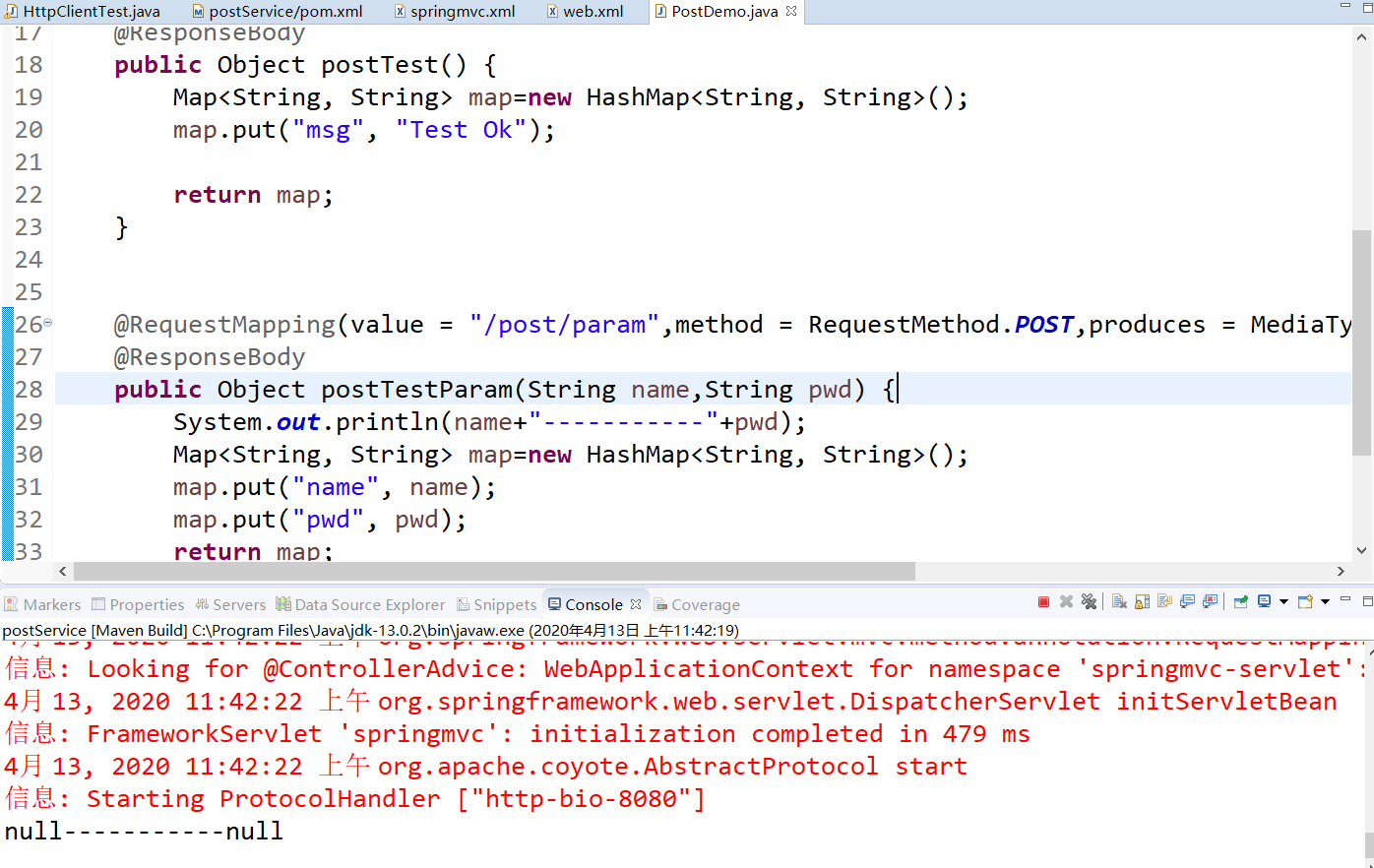
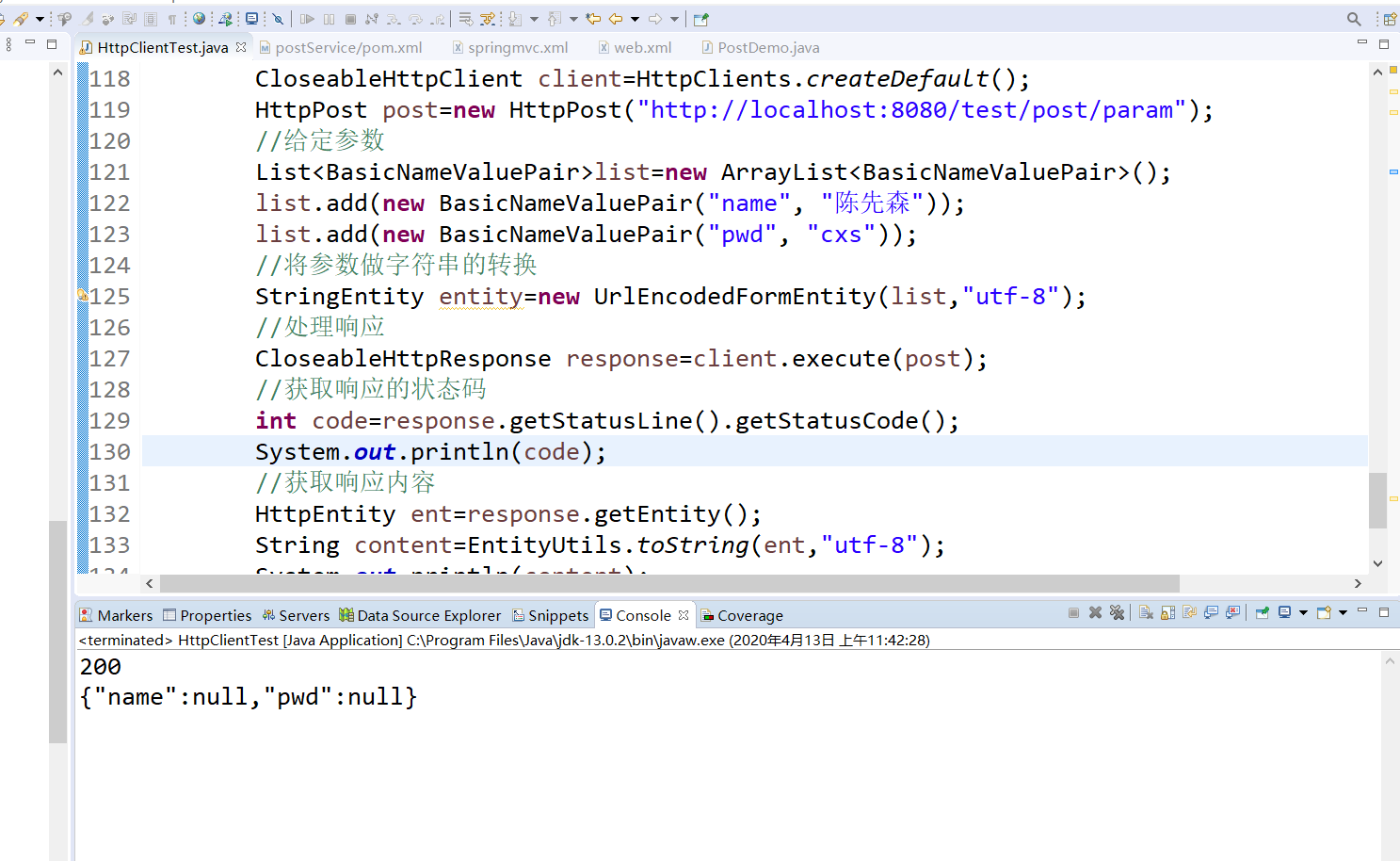
package com.bjsxt.web.controller;
import java.util.HashMap;
import java.util.Map;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
@RequestMapping("/test")
public class PostDemo {
@RequestMapping(value = "/post",method = RequestMethod.POST)
@ResponseBody
public Object postTest() {
Map<String, String> map=new HashMap<String, String>();
map.put("msg", "Test Ok");
return map;
}
@RequestMapping(value = "/post/param",method = RequestMethod.POST,produces = MediaType.APPLICATION_JSON_VALUE+";chaeset=utf-8")
@ResponseBody
public Object postTestParam(String name,String pwd) {
System.out.println(name+"-----------"+pwd);
Map<String, String> map=new HashMap<String, String>();
map.put("name", name);
map.put("pwd", pwd);
return map;
}
}
package com.bjsxt.httpclient.test;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import javax.sound.midi.VoiceStatus;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public class HttpClientTest {
public static void main(String[] args) throws Exception {
// TODO Auto-generated method stub
// HttpClientTest.doGet();
// HttpClientTest.doGetParam();
// HttpClientTest.dePostTest();
HttpClientTest.doPostParamTest();
}
/**
* get请求不带参数
*
* @throws IOException
* @throws ClientProtocolException
*/
public static void doGet() throws ClientProtocolException, IOException {
// 创建一个HttpClient对象
CloseableHttpClient client = HttpClients.createDefault();
// 创建get请求对象,在请求中输入url
HttpGet get = new HttpGet("http://baidu.com");
// 发送请求,并返回响应
CloseableHttpResponse response = client.execute(get);
// 处理响应
// 获取响应的状态码
int code = response.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = response.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
// 关闭链接
client.close();
}
/**
* get请求带参数
*
* @throws URISyntaxException
* @throws IOException
* @throws ClientProtocolException
*/
public static void doGetParam() throws URISyntaxException, ClientProtocolException, IOException {
CloseableHttpClient client = HttpClients.createDefault();
// 创建一个封装url的对象,在该对象中可以给定请求参数
URIBuilder builder = new URIBuilder("https://www.sogou.com/web");
builder.addParameter("query", "西游记");
// 创建一个get请求对象
HttpGet get = new HttpGet(builder.build());
// 发送请求,并返回响应
CloseableHttpResponse response = client.execute(get);
// 处理响应
// 获取响应的状态码
int code = response.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = response.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
// 关闭链接
client.close();
}
/**
* post请求不带参数
*
* @throws IOException
* @throws ClientProtocolException
*/
public static void dePostTest() throws ClientProtocolException, IOException {
CloseableHttpClient client = HttpClients.createDefault();
HttpPost post = new HttpPost("http://localhost:8080/test/post");
// 发送请求,并返回响应
CloseableHttpResponse response = client.execute(post);
// 处理响应
// 获取响应的状态码
int code = response.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = response.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
// 关闭链接
client.close();
}
/**
* post请求带参数
*
* @throws Exception
*/
public static void doPostParamTest() throws Exception {
CloseableHttpClient client=HttpClients.createDefault();
HttpPost post=new HttpPost("http://localhost:8080/test/post/param");
//给定参数
List<BasicNameValuePair>list=new ArrayList<BasicNameValuePair>();
list.add(new BasicNameValuePair("name", "陈先森"));
list.add(new BasicNameValuePair("pwd", "cxs"));
//将参数做字符串的转换
StringEntity entity=new UrlEncodedFormEntity(list,"utf-8");
//处理响应
CloseableHttpResponse response=client.execute(post);
//获取响应的状态码
int code=response.getStatusLine().getStatusCode();
System.out.println(code);
//获取响应内容
HttpEntity ent=response.getEntity();
String content=EntityUtils.toString(ent,"utf-8");
System.out.println(content);
//关闭链接
client.close();
}
}