// pages/food/food.js
var productData = require('../../utils/productData.js');
var request = require('../../utils/request.js')
Page({
/**
* 页面的初始数据
*/
data: {
location: '',
productType: [],
listdata: [],
num: 1,
latitude:'',
longitude:''
},
/**
* 生命周期函数--监听页面加载
*/
onLoad: function (options) {
this.setData({
productType: productData
});
//进入食疗方 获取定位
wx.getLocation({
success: res=>{
console.log(res.latitude);
console.log(res.longitude);
this.setData({
latitude: res.latitude,
longitude:res.longitude
});
wx.request({
url: 'http://iwenwiki.com:3002/api/lbs/location',
data: {
latitude: this.data.latitude,
longitude: this.data.longitude
},
success: result => {
console.log(result.data.result.address_component.city);
var cityName = result.data.result.address_component.city.slice(0,2);
this.setData({
location:cityName
});
console.log(this.data.location);
}
})
},
fail: err => {
console.log(err);
}
});
console.log(this.data.location);
// console.log(productData);
var data1 = {
city: this.data.location,
page: this.data.num
}
request('get', '/api/foods/list', data1, true, (res) => {
console.log(res.data.result);
this.setData({
listdata: res.data.result
})
}, function (error) {
console.log(error);
}, function () {
console.log('已经没有数据可以加载了');
})
// console.log(request);
// 获取食疗方数据
// wx.request({
// url: 'http://iwenwiki.com:3002/api/foods/list',
// data:{
// city:this.data.location,
// page:this.data.num
// },
// success:res=>{
// console.log(res.data.data);
// wx.showLoading({
// title: '加载中',
// })
// if(res.data.status==200){
// console.log(res.data.data.result);
// this.setData({
// listdata:res.data.data.result,
// isShow:true
// })
// }
// },
// complete:res=>{
// wx.hideLoading()
// }
// })
},
// 点击分类,进入相对应页面
productType: function (e) {
console.log(e);
wx.navigateTo({
url: '../productType/productType?ID=' + e.currentTarget.dataset.mark,
})
},
// 按钮--加载更多数据
getMore: function () {
this.data.num++;
console.log(this.data.num);
request('get', '/api/foods/list', data1, true, (res) => {
console.log(res.data.result);
this.setData({
listdata: this.data.listdata.concat(res.data.result),
});
this.setData({
isShow: true
})
}, (error) => {
console.log(error);
},
function () {
this.setData({
isShow: false
})
console.log('已经没有数据可以加载了');
})
//----------------------------
// wx.request({
// url: 'http://iwenwiki.com:3002/api/foods/list',
// data:{
// city:this.data.location,
// page:this.data.num,
// isShow:false
// },
// success:res=>{
// console.log(res.data.data);
// wx.showLoading({
// title: '加载中',
// })
// if(res.data.status==200){
// console.log(res.data.data.result);
// this.setData({
// listdata:this.data.listdata.concat(res.data.data.result),
// })
// }else{
// this.setData({
// isShow:false
// })
// console.log('没有数据了,加载完了');
// }
// },
// complete:res=>{
// wx.hideLoading()
// }
// })
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady: function () {
},
/**
* 生命周期函数--监听页面显示
*/
onShow: function () {
console.log(11);
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide: function () {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload: function () {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
//下拉刷新
onPullDownRefresh: function () {
this.setData({
num: 1
})
var data1 = {
city: this.data.location,
page: this.data.num
}
request('get', '/api/foods/list', data1, true, (res) => {
console.log(res.data.result);
this.setData({
listdata: res.data.result
})
}, function (error) {
console.log(error);
}, function () {
console.log('已经没有数据可以加载了');
})
//----------------------------------------------
// wx.request({
// url: 'http://iwenwiki.com:3002/api/foods/list',
// data:{
// city:this.data.location,
// page:this.data.num
// },
// success:res=>{
// console.log(res.data.data);
// wx.showLoading({
// title: '加载中',
// })
// if(res.data.status==200){
// console.log(res.data.data.result);
// this.setData({
// listdata:res.data.data.result,
// // isShow:true
// })
// }
// },
// complete:res=>{
// wx.hideLoading()
// }
// })
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom: function () {
//下拉加载更多
this.data.num++;
console.log('下拉到底了');
var data1 = {
city: this.data.location,
page: this.data.num,
}
request('get', '/api/foods/list', data1, true, (res) => {
console.log(res.data.result);
this.setData({
listdata: this.data.listdata.concat(res.data.result),
})
}, (error) => {
console.log(error);
},
function () {
console.log('已经没有数据可以加载了');
})
// wx.request({
// url: 'http://iwenwiki.com:3002/api/foods/list',
// data:{
// city:this.data.location,
// page:this.data.num,
// isShow:false
// },
// success:res=>{
// console.log(res.data.data);
// wx.showLoading({
// title: '加载中',
// })
// if(res.data.status==200){
// console.log(res.data.data.result);
// this.setData({
// listdata:this.data.listdata.concat(res.data.data.result),
// })
// }else{
// this.setData({
// isShow:false
// })
// console.log('没有数据了,加载完了');
// }
// },
// complete:res=>{
// wx.hideLoading()
// }
// })
},
/**
* 用户点击右上角分享
*/
onShareAppMessage: function () {
}
})
老师 视频讲课时一开始进入食疗方页面是默认北京的现在改成一进入食疗方这个页面就获取当前城市 我看后台输出data数据已经改成了当前城市 当时那个请求数据列表的请求没返回任何东西也没报错
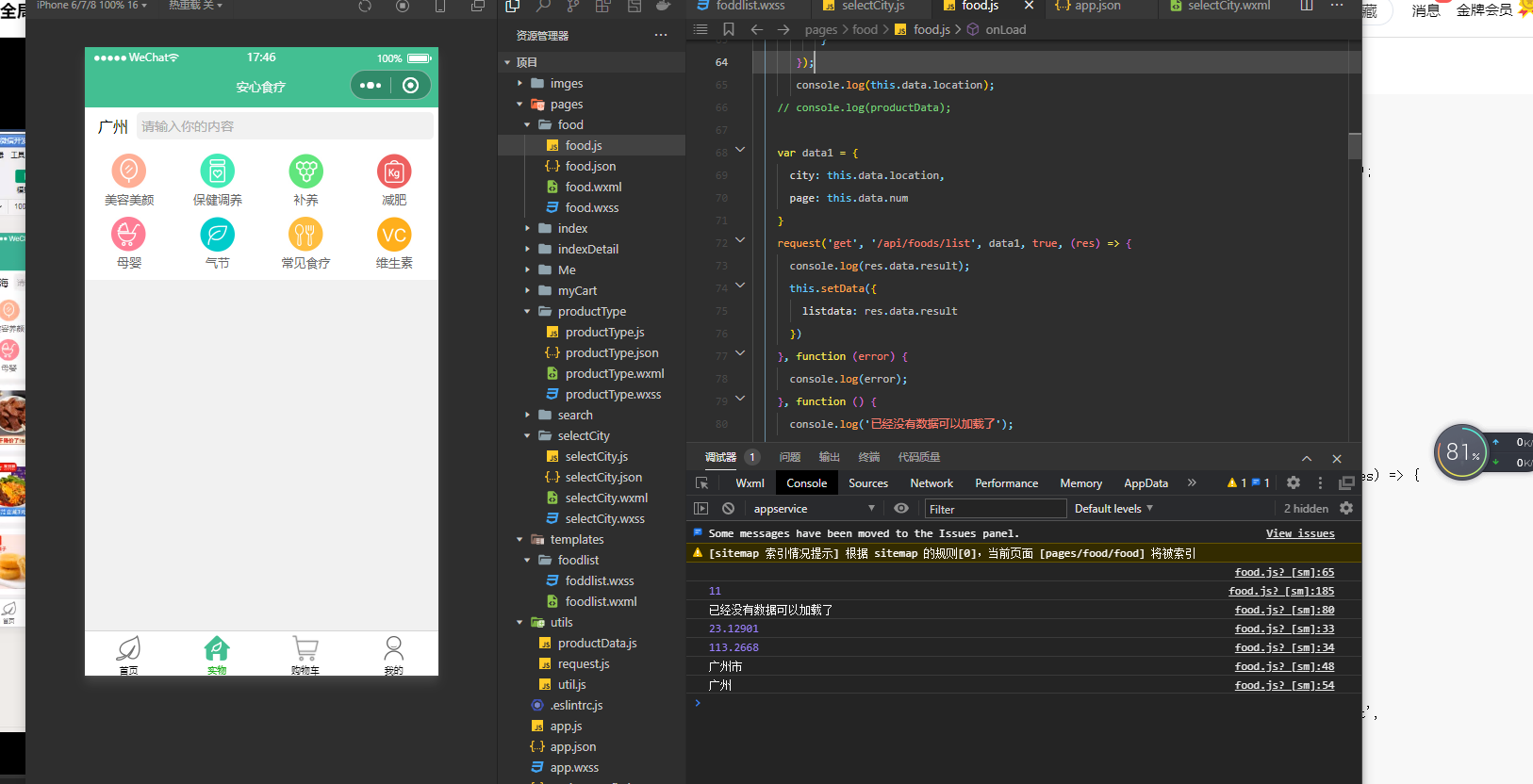