from tkinter import *
from tkinter import messagebox
import webbrowser
class Application(Frame):
def __init__(self, master=None):
super().__init__(master) # super()代表的是父类的定义, 而不是父类对象
self.master = master
self.pack()
self.createWidget()
def createWidget(self):
self.w1 = Text(root, width=40, height=12, bg='gray')
self.w1.pack()
self.w1.insert(1.0, '2021年度电脑销量排名\n') # 行从1开始,列从0开始
self.w1.insert(2.0, '自己问度娘去')
# side=left 表示左排列,不然居中排列
Button(self, text="重复插入文本", command=self.insertText).pack(side='left')
Button(self, text='返回文本', command=self.returnText).pack(side='left')
Button(self, text='添加图片', command=self.addImage).pack(side='left')
Button(self, text='添加组件', command=self.addWidget).pack(side='left')
Button(self, text='通过tag精确控制文本', command=self.textTag).pack(side='left')
def insertText(self):
# INSERT索引表示在光标处插入
self.w1.insert(INSERT, 'Lenovo')
# END 索引表示在最后插入
self.w1.insert(END, 'computer')
def returnText(self):
# Index(索引)是用来指向 Text 组件中文本的位置,Text 的组件索引也是对应实际字符之间的位置
# 核心:行号以 1 开始,列号以 0 开始
print(self.w1.get(1.2, 1.6))
print('所有文本内容:\n' + self.w1.get(1.0, END))
def addImage(self):
global photo
photo = PhotoImage(file="logo.gif")
self.w1.image_create(END, image=photo)
def addWidget(self):
b1 = Button(self.w1, text="爱尚学堂") # 在Text创建组建的命令
self.w1.window_create(INSERT, window=b1)
def textTag(self):
self.w1.delete(1.0, END) # 删除全部文本
self.w1.insert(INSERT, 'good good study,day day up!\n北京尚学堂\n百战程序员\nbaidu')
self.w1.tag_add('good', 1.0, 1.9)
self.w1.tag_configure("good", background="yellow", foreground="red")
# 添加标记,标记区域为第四行的第0-2列
self.w1.tag_add("baidu", 4.0, 4.2)
self.w1.tag_configure("baidu", underline=True) # 添加下划线
self.w1.tag_bind("baidu", "<Button-1>", self.webshow) # 绑定事件
def webshow(self, event): # 绑定事件 打开百度页面
webbrowser.open("http://www.baidu.com")
if __name__ == '__main__':
root = Tk()
root.geometry("500x300+300+300")
root.title("欢迎光临")
app = Application(master=root)
root.mainloop()
你好 老师 两个问题:
为啥我出来的只有ba两个字母下面有下划线 不是整个baidu下划线
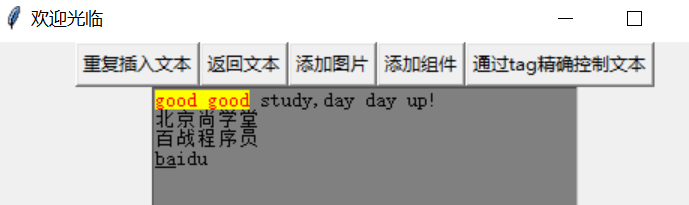
添加图片一次有效,再点击添加图片 上一次添加的就不见了 这是为啥 谢谢。